PROJECT: Student Schedule Planner
1. Introduction
This project portfolio showcases a collection of software engineering projects I have worked on during my time studying in NUS.
The first project in this portfolio is Student Schedule Planner, a desktop schedule planner application created by my team T12-3 for the CS2103T module. This application is targeted at university students for keeping track of their tasks. We were tasked to morph it from an existing product called AddressBook, which had around 10,000 lines of existing code. We also had to work under multiple constraints such as the primary mode of user input must be through command line.
My key contributions in this project were mainly improvements to the UI. The display is updated accordingly whenever a user makes changes to the schedule planner.
2. Summary of Contributions
-
Major enhancement: Major redesign of GUI
-
What it does: The app displays progress bars, categories, and tags.
-
Justification: This feature improves user experience because the user will be able to tell how much work he/she has completed and what categories and tags he/she has used.
-
Highlights: The implementation was challenging as it required learning how to code for JavaFX.
-
Credits: CSS styling for progress bars
-
-
Minor enhancements:
-
Added
listarchived
command that displays all of the archived tasks -
Added
progresstoday
andprogressweek
commands to display the exact percentage of user’s progress
-
-
Code contributed: [RepoSense]
-
Other contributions:
-
Enhancements to existing features:
-
Documentation:
-
Corrected typos, grammatical errors and inconsistent uses of language in User Guide and Developer Guide
-
-
Community:
-
3. Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation in terms that are not too technical so that end-users without technical knowledge can understand. |
3.1. Viewing Archived Tasks
List all archived tasks.
listarchived
Example:
Step 1: archive 1
Below is the screenshot of task list that will appear on screen after step 1.
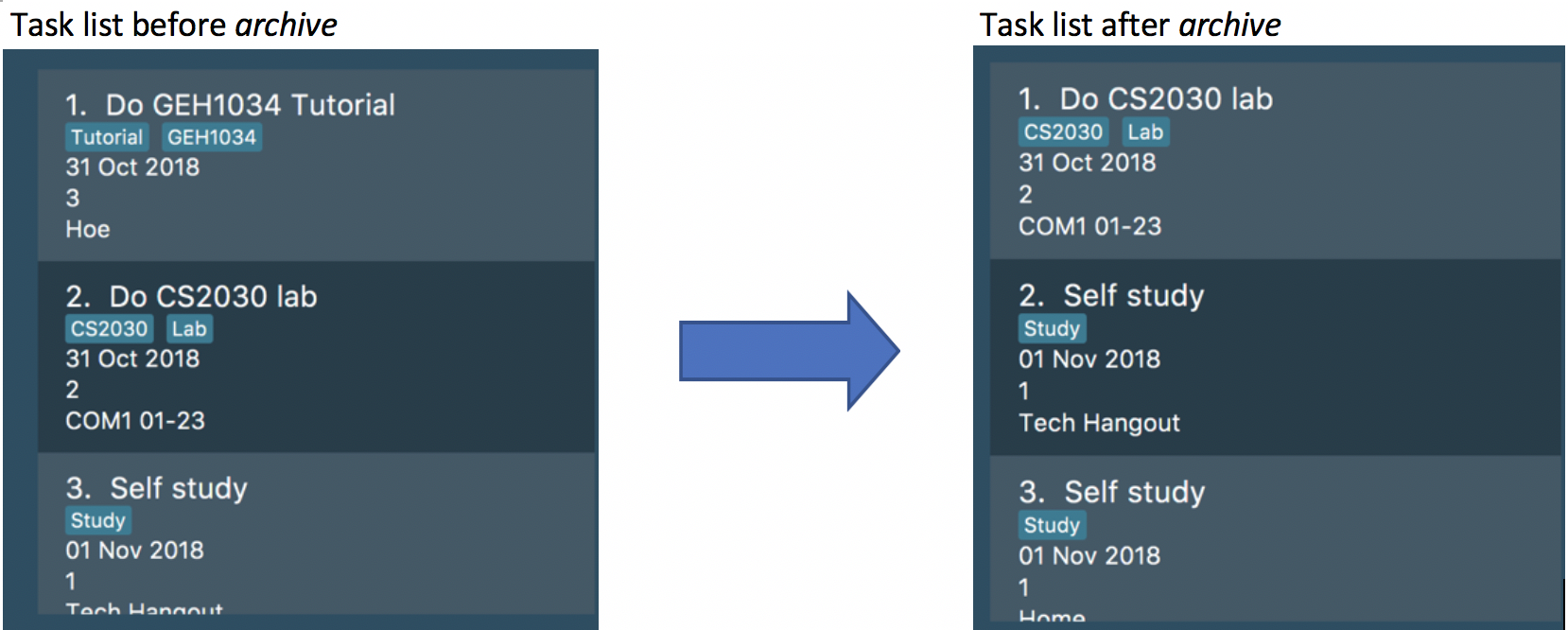
Step 2: listarchived
Below is the screenshot of archived task list that will appear on screen after step 2.
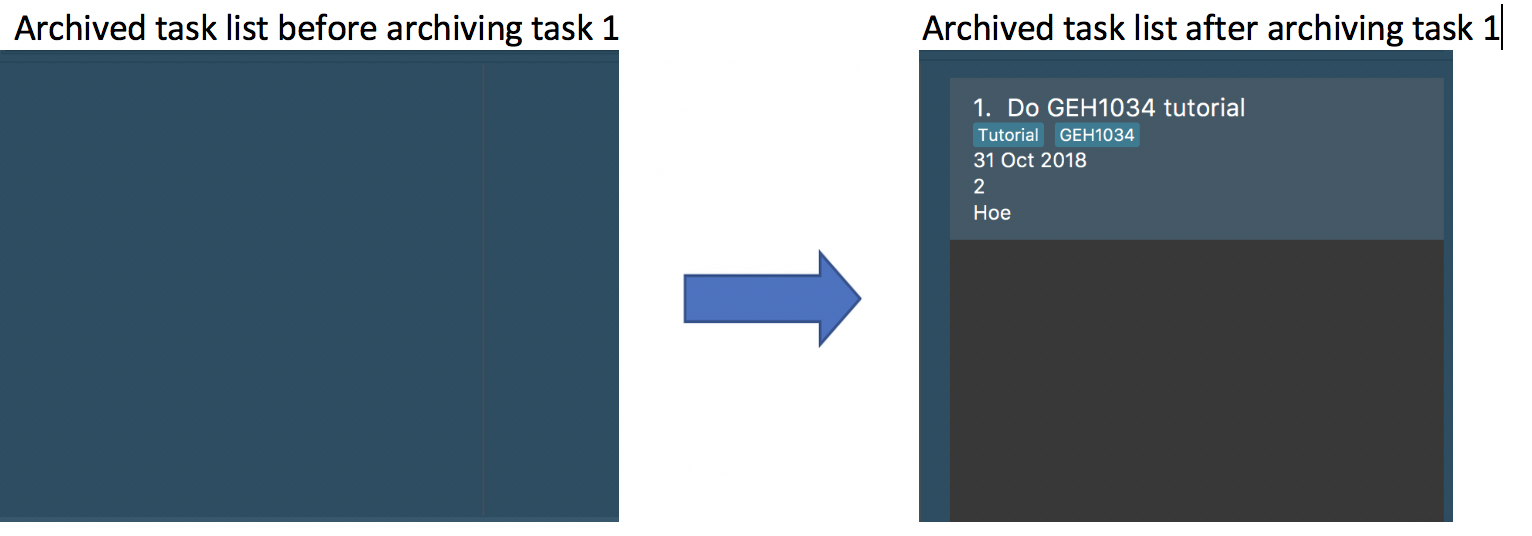
3.2. Displaying Category Tags
Show all tags categorised under the specified category. It expands the tab in the sidebar.
tags c/CATEGORY
Example:
tags c/Modules
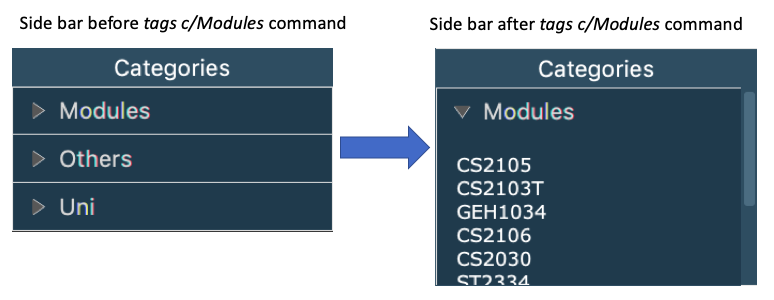
3.3. Displaying Today’s Progress
Show the percentage of tasks archived for the day in the command result box and lists the uncompleted tasks for today
. The progress bar is also displayed at the bottom left of the window.
progresstoday
Example:
Step 1: listday
Step 2: archive 1
Step 3: progresstoday
The diagram below illustrates the SSP after each of the commands entered above.
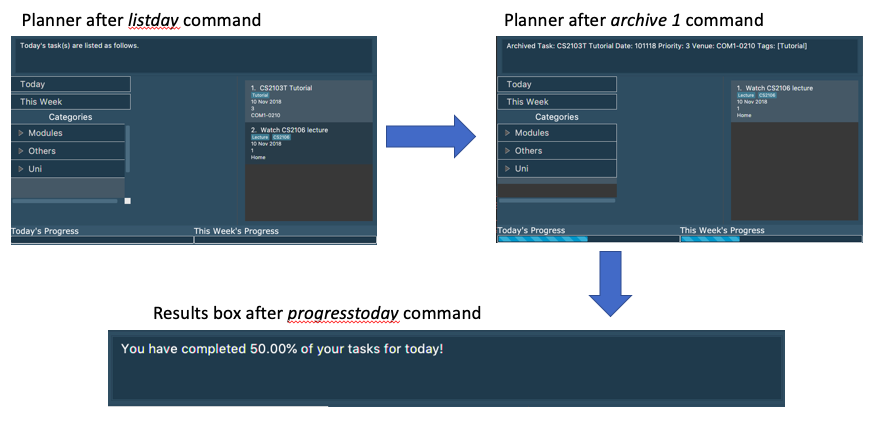
3.4. Displaying This Week’s Progress
Show the percentage of tasks archived from today to the nearest Sunday in the command result box, and lists the
uncompleted tasks from today until the nearest Sunday. The progress bar is also displayed at the bottom right of the
window.
progressweek
Example:
Step 1: listday
Step 2: archive 1
Step 3: progressweek
The diagram below illustrates the SSP after each of the commands entered above.
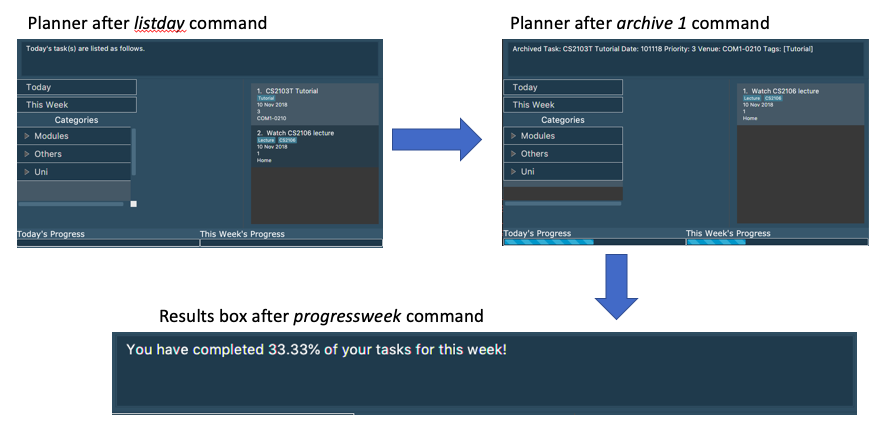
4. Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. It also shows my ability to explain how my implementations work so that future coders that work with my code will be able to improve my code easily. |
4.1. List Archived Tasks
The listarchived
command allows the user to view the list of all archived tasks. Whenever the user completes a task,
he/she will archive it. When archived, the task will be moved from the task list into a separate archive list that stores
all completed tasks. If the user wishes to view all of his/her completed tasks, the listarchived
command will display
all of the archived tasks.
4.1.1. Current Implementation
Model contains two UniqueTaskLists - tasks and archivedTasks - each containing the tasks and archived tasks respectively.
Given below is an example usage scenario and how the list archived mechanism behaves at each step:
Step 1. The user executes the command listarchived
.
Step 2. The listarchived
command raises a new ChangeViewEvent that signals a change to archived view.
Step 3. MainWindow responds to the ChangeViewEvent with MainWindow#handleChangeViewEvent().
Step 4. MainWindow calls Logic#getFilteredArchivedTaskList(). It then creates a new TaskListPanel instance with Tasks in that list.
Step 5. MainWindow places the new TaskListPanel in the TaskListPanelPlaceHolder. The archived tasks are now displayed.
The following sequence diagram shows how the list archive operation works:
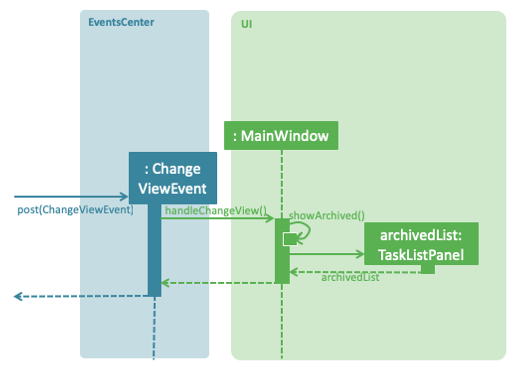
4.1.2. Design Considerations
Aspect: How to change what is displayed
-
Alternative 1 (current choice): The task list panel’s contents are replaced with a new task list containing archived tasks.
-
Pros: Only one section of the UI has to be changed.
-
-
Alternative 2: Two JavaFX scenes are created. To handle display changes, switch to the corresponding scene.
-
Pros: It is the proper way of handling change in display with JavaFX.
-
Cons: Since only one section of the UI needs to be changed, changing the whole scene seems redundant.
-
4.2. Display Tags in Sidebar
Whenever the user tags a task with a new tag, the tag will be added to the sidebar panel. The user will be able to view all of the tags he/she has used to categorize tasks. Entering the command tags c/CATEGORY will expand the tab for the specified category to show all tags listed under that category.
4.2.1. Current Implementation
Every time any category or tag is changed in the Student Schedule Planner, it raises a SchedulePlannerChangedEvent. The UI will then handle that event and update the side bar panel with the new version of categories and tags.
Given below is an example usage scenario and how the schedule planner behaves at each step:
Step 1. The user executes command addtag c/Modules t/CS2100
.
Step 2. The addtag
command updates the model with the new tag and raises a new SchedulePlannerChangedEvent.
Step 3. SidebarPanel responds to the SchedulePlannerChangedEvent with SidebarPanel#handleSchedulePlannerChangedEvent().
Step 4. The Accordion containing all the categories and tags is cleared.
Step 5. The Accordion is filled with the new list of updated categories and tags.
Step 6. The user executes command tags c/Modules
.
Step 7. The ShowTagsCommand raises a new ShowTagsRequestEvent("Modules").
Step 8. SidebarPanel responds to the ShowTagsRequestEvent with SidebarPanel#handleShowTagsRequestEvent().
Step 9. The TitledPane with the matching name "Modules" is expanded to display all the tags listed under the Modules category.
Below is a code snippet showing how the SidebarPanel handles a ShowTagsRequestEvent:
@Subscribe public void handleShowTagsRequestEvent(ShowTagsRequestEvent e) { String catName = e.getCategory(); ObservableList<TitledPane> titledPanes = accordion.getPanes(); for (TitledPane titledPane : titledPanes) { if (titledPane.getText().equals(catName)) { accordion.setExpandedPane(titledPane); } } }
4.2.2. Design Considerations
Aspect: How to update the tags on UI
-
Alternative 1 (current choice): Clear all the TitledPanes and add new TitledPanes accordingly.
-
Pros: No matter which category or tag is changed, or what type of change (ie. delete, add, or edit), this change can be handled by the same method each time.
-
Cons: It is redundant to clear everything and replace them with new TitledPanes.
-
-
Alternative 2: Handle different kinds of changes to the category or tag lists.
-
Pros: It is a lot faster to only change the TitledPane that is affected.
-
Cons: There are too many cases for how the lists can be changed. (ie. a different change is needed for each of these cases: category is deleted/edited/created/cleared, or a tag is deleted/added)
-
4.3. Display Progress Bars
The progress bars for today’s progress and this week’s progress at the bottom reflect the percentages automatically when the schedule planner is changed in any way.
4.3.1. Current Implementation
Whenever the user makes a change in the schedule planner, for example add, delete, or archive a task, it raises a new SchedulePlannerChangedEvent. UI part ProgressBarPanel will handle this event and update the values for both today and this week’s progress bars.
Given below is an example usage scenario and how the progress bar mechanism behaves at each step:
Step 1. User archives a Task due today.
Step 2. A new SchedulePlannerChangedEvent is raised.
Step 3. ProgressBarPanel handles the event with ProgressBarPanel#handleSchedulePlannerChangedEvent().
Step 4. The taskList is retrieved by SchedulePlannerChangedEvent#data.getTaskList(). The archivedTaskList is retrieved by SchedulePlannerChangedEvent#data.getArchivedTaskList().
Step 5. ProgressBarPanel#updateProgressBars(taskList, archivedTaskList) is called.
Step 6. After filtering through each lists with the DateSamePredicate, their sizes are calculated.
Step 7. The number of completed tasks for today is the size of the filtered archived list, while the total number of tasks for today is the size of both the filtered archived list and the filtered task list.
Step 8. The fraction is calculated from completed / total. Then the progress bar for today is set to that fraction.
Step 9. The same is done for this week’s progress bar but the lists are filtered with DateWeekSamePredicate.
The following sequence diagram shows how the progress bar mechanism works:
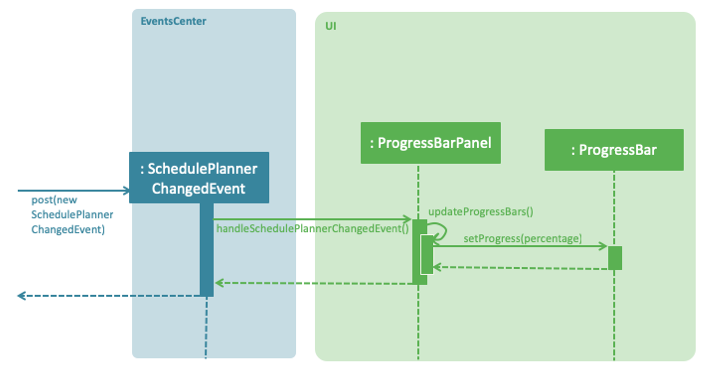
4.3.2. Design Considerations
Aspect: How the percentages are calculated
-
Alternative 1 (current choice): Calculate the percentage of progress by finding the size of filtered task list and filtered archived list.
-
Pros: It is easy to implement.
-
Cons: It may be slow if the task list and archived task list are very long.
-
-
Alternative 2: Keep track of number of uncompleted and completed tasks in new Objects called Day and Week.
-
Pros: It is much faster to calculate the percentage compared to filtering each task list by a predicate then calculating its size.
-
Cons: The Day and Week objects must be updated for a majority of the commands the app supports. If I had more time, I would have been able to implement this fully.
-