PROJECT: Student Schedule Planner
1. Introduction
This project portfolio showcases my contributions to Student Schedule Planner, and how I implemented each of the features.
In this project, I, together with Chen Shin, Lin Xu, Thaddeus Lim and Xiao Yilin, morphed a generic Address Book application into a specialised Student Schedule Planner aimed exclusively at university students. This was done with the constraint that a Command Line Interface (CLI) was to be the main mode of interaction with the application.
As mentioned before, Student Schedule Planner targets a very specific group of people: students. We took into account the pains that we as university students faced when we were using conventional to-do lists and aimed to solve these pains during this project.
Student Schedule Planner is hence a desktop application made specifically for students. The user interacts with the application using a CLI, but it has a Graphical User Interface (GUI) for users to view results in a more user-friendly manner. The application was built using Java and has approximately 10 kLoC.
2. Summary of contributions
-
Major enhancement: added the ability to add recurring tasks
-
What it does: allows the user to add a recurring task by specifying the number of repeats and interval between each repeat.
-
Justification: This feature gives convenience to users as users with to-do tasks that happen repeatedly (eg. weekly) will not have to manually add the same tasks over and over again.
-
Highlights: The implementation forced me to come up with new field classes. The implementation required an understanding of the Calendar API, and duplicate tasks had to be handled.
-
Credits: The Calendar API from Java provided a variety of functions made implementing the AddRepeatCommand simpler than if I had started from scratch.
-
-
Minor enhancement: added a list overdue command that allows the user to view his/her overdue tasks.
-
Code contributed: [RepoSense]
-
Other contributions:
3. Contributions to the User Guide
Below are my contributions to the User Guide. This shows my ability to write effective documentation for the features I implemented. Furthermore, I helped to refactor the initial user guide for address book level 4 into a user guide for our current application, Student Schedule Planner. |
3.1. Adding a Recurring Task
Add tasks that occur in regular intervals.
repeat r/REPEATS i/INTERVAL n/NAME p/PRIORITYLEVEL t/[TAG] d/DEADLINE v/VENUE
Example:
repeat r/4 i/7 n/CS2103T Tutorial p/3 t/Tutorial d/111018 v/COM1-0210
Adds 4 tasks named CS2103T Tutorial
with priority level 3
,
tag Tutorial
, venue at COM1-0210
, and deadlines on the 11th October 2018, 18th October 2018, 25th October 2018, and 1st November 2018.
The diagram below illustrates the SSP before and after the above command was entered:
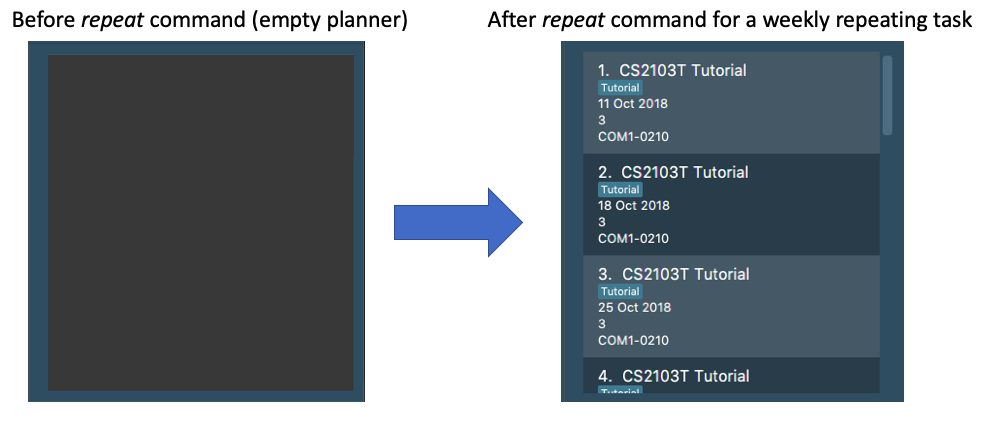
The number of repeats should be a positive integer (≥1). Setting the repeat as 1 has the same
effect as using the add command.
The number of repeats should be an integer that is greater or equals to 1. Setting the repeat as 1 has the same effect as using the add command.
|
Use this command to schedule tasks that you carry out regularly. |
The maximum number of repetitions is 15. |
Tasks whose deadlines go beyond 2099 will have their deadlines set to the 21st century instead. For example, a task created by the AddRepeatCommand that with the deadline on 1st January 2101 will instead have the deadline set to 1st January 2001 instead. |
3.2. Viewing Overdue Tasks
Listing all the overdue tasks.
listoverdue
Example:
listoverdue
(on the date 111118)
Lists tasks due on 101118 and before.
The following diagram illustrates the SSP before and after listoverdue
was entered:
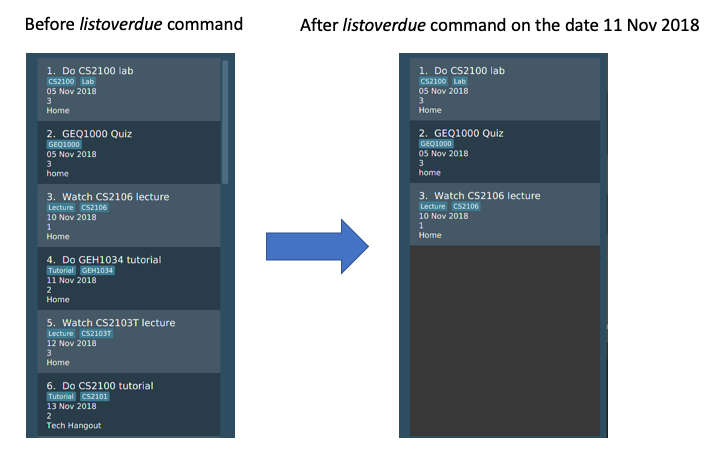
4. Contributions to the Developer Guide
_Below are my contributions to the Developer Guide. This showcases the technical depth of my contributions to the application and my ability to make abstract technical information easier to understand. This will allow future developers to change or expand on my existing code effortlessly. |
4.1. Add Recurring Tasks
The Add Repeat feature allows the user to add recurring tasks of a specified number of repeats and a specified interval between each repeat.
4.1.1. Current Implementation
The following code snippet shows how the command is implemented:
... // Loop through to add the rest of the tasks. for (int i = 1; i < Integer.parseInt(repeat.value); i++) { baseDate.add(Calendar.DAY_OF_YEAR, interval); newDate = schedulerFormat.format(baseDate.getTime()); date = new Date(newDate); newTask = new Task(toAdd.getName(), date, toAdd.getPriority(), toAdd.getVenue(), toAdd.getTags()); // Add the task only if there is no duplicate task within the model. if (!model.hasTask(newTask)) { model.addTask(newTask); } } ...
This loop occurs after the first task is added. The tasks are created within the loop with duplicate names, priorities, venues and tags. Before adding a task, the function checks with the model if such a task already exists. If not, the task is added. If the task is a duplicate task, it is skipped and the loop proceeds until it terminates.
The following outlines an example of the command’s usage.
Step 1. The user enters the command repeat n/CS2103T Tutorial i/7 r/3 p/3 t/CS2103T t/Tutorial v/COM1
Step 2. The AddRepeatParser parses the command and isolates each of the arguments.
Step 3. The Model checks if the first task exists. If the first task does not exist in Model, it is added. If not, it is skipped.
Step 4. The execute command loops through to build each repeated task. In each loop, the date is incremented by the specified interval. Same as Step 3, a duplicate task is skipped and a task that does not exist in Model is added.
Step 5. The changes are committed and the new list is shown to the user.
The following sequence diagram shows how the add repeat operation works:
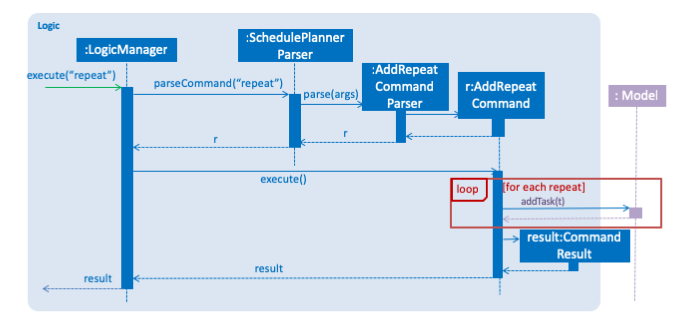
4.2. List Overdue Tasks
The listoverdue
command allows the user to view the list of all overdue tasks. A task is considered overdue if its
deadline has passed. In other words, the system’s current date is after the deadline.
4.2.1. Current Implementation
Given below is a code snippet of the ListOverdueCommand.
... public CommandResult execute(Model model, CommandHistory history) { // Filter the task list using the OverduePredicate with the current date as the parameter. model.updateFilteredTaskList(new OverduePredicate(SYSTEM_DATE)); EventsCenter.getInstance().post(new ChangeViewEvent(ChangeViewEvent.View.NORMAL)); return new CommandResult(MESSAGE_SUCCESS); } ...
The ListOverdueCommand calls the method updateFilteredTaskList with a new OverduePredicate which has the current date as the parameter.
public boolean test(Task task) { return date - task.getDate().yymmdd > 0 ? true : false; }
The test function within OverduePredicate class compares the current system date with the date of the task. If the task’s date is after the current time, the function returns false, and vice versa.
Model contains a UniqueTaskList called tasks.
Given below is an example usage scenario and how the list overdue mechanism behaves at each step:
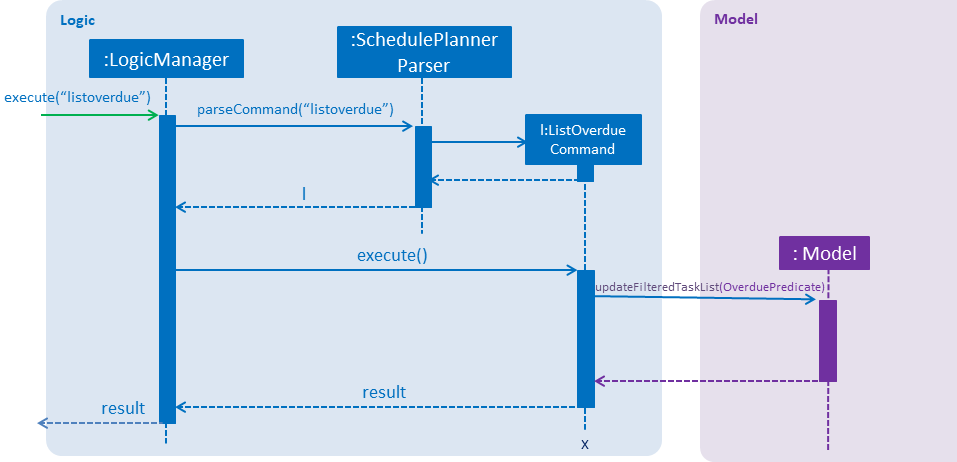
Step 1. The user executes the command listoverdue
.
Step 2. model.updateFilteredTaskList()
will update the task list with OverduePredicate
as the parameter
OverduePredicate
itself takes the current system date in the yyMMdd format.
Step 3. The updated task list would be reflected on the UI to be displayed to the user.
4.2.2. Design Considerations
Aspect: How the overdue tasks are stored
-
Alternative 1 (current choice): The overdue tasks are not stored in a separate UniqueTaskList. Instead, the UniqueTaskList tasks is filtered with OverduePredicate each time the
listoverdue
command is called.-
Pros: Does not require a new UniqueTaskList.
-
Cons: If the number of tasks is huge, filtering will take a long time. However, this is not a problem since the number of tasks is small at any given point of time.
-
-
Alternative 2: The overdue tasks are stored in a new UniqueTaskList.
-
Pros: Retrieving is overdue tasks is faster.
-
Cons: A new UniqueTaskList has to be implemented.
-
5. PROJECT:
Prior to this project, I have undertaken various software engineering projects, either for Orbital 2018 or for hackathons.
This Android-based application allows users to practice their speaking skills by helping them to quantify their speaking speed and user of various filler words.
This Telegram bot was built to help researchers and students to effortlessly summarise articles and research papers and generate a bibligraphy in either the APA or MLA format. It helps users to compare a list of articles to see how similar they are.