Overview
This Personal Project Portfolio details the contributions which I have made to the Student Schedule Planner(SSP) project. It also outlines skills which I have picked up.
Student Schedule Planner (SSP) is a desktop application, created by Team T12-3. It is a planner customised for university students, and it aims to help university students manage their time effectively.
SSP incorporates various features that streamline the creation, organisation, and finding of tasks. In addition, it allows the user to keep track of the current academic week, and has a progress tracking feature, which displays user progress.
Instead of utilising a conventional Graphical User Interface(GUI). SSP is optimised for users who prefer typing out their commands. This application has the potential to be much faster than regular planner applications, especially for users who are proficient in typing.
Summary of contributions
In total, I contributed approximately 1500+ lines of code, in both features and documentation. The features I
implemented were listmonth
, filter
, and filterstrict
.
I also aided in refactoring major parts of the codebase, morphing the original "Address Book (Level 4)" code to suit our new SSP application. This included changing "addressbook" and "person" references in the code to "scheduleplanner" and "task" references respectively.
The features I implemented are as follows:
Major enhancements:
-
Ability to list remaining number of tasks in the current month
-
What it does: This feature allows the user to list all remaining tasks in the current month, in chronological order. This feature takes into account leap years.
-
Justification: The user can narrow the scope of the list to the current month, making it easier to manage tasks within this period. This provides a convenient way to list tasks that occur in commonly used time frames(day, week, month).
-
Highlights: It required an in-depth analysis of design alternatives. The implementation was challenging as it had to factor in the variations of days between months, and in February during leap years.
-
Credits: Code from ListWeekCommand was referenced in the implementation of ListMonthCommand
-
-
Ability to filter tasks inclusively according to tags
-
What it does: This feature allows the user to filter tasks inclusively by their tags. This means that given 2 or more tags as user-input, tasks with ANY of the specified tags will be listed. This includes any number of tags.
-
Justification: The user can filter out tasks with certain tags, expediting the search process, and reducing the time needed to find a specific task, or tasks belonging to the same category.
-
Highlights: The implementation was challenging, as it required the filter feature to be able to search for more multiple tags. This was made complicated by the fact that each task may contain multiple tags
-
Credits: Code from FindCommand was referenced in the implementation of FilterCommand
-
-
Ability to filter tasks exclusively according to tags
-
What it does: This feature allows the user to filter tasks exclusively by their tags. This means that given 2 or more tags to filter, only tasks with ALL of the specified tags will be listed.
-
Justification: The user can now narrow the scope of the filter function, further expediting the search process. This feature caters to users who prefer a more strict filter mechanic.
-
Highlights: The implementation made small tweaks to the filter predicate such that, only tasks containing tags that match all user-input tags will be displayed.
-
Credits: Code from FilterCommand was referenced in the implementation of FilterCommand
-
Minor enhancements:
-
Code contributed: RepoSense
-
Other contributions:
-
Project management:
-
Documentation:
-
Enhanced Introduction for DevGuide: #77
-
Added Use Cases for DevGuide: #115
-
Added Instructions for Manual Testing in Developer Guide: #251
-
Improvements to flow of existing contents of the User Guide: #64 , #140, #245 ,
-
Improvements to flow of existing contents of the Developer Guide: #64 , #87 , #221 , #224 , #245
-
-
Community:
-
Contributions to the User Guide
Provided below are the sections I have contributed to the User Guide. They showcase my ability to write concise, and coherent documentation targeting end-users who are seeing the SSP application for the first time |
Viewing Tasks Due This Month
List tasks due from current date till the end of the current Month.
listmonth
Example:
listmonth
(on the date 111118)
Tasks from 111118 to end of the month (301118) are listed.
The following diagram illusatrates the SSP when listmonth
is executed on the date 111118
. Only tasks until
301118
, which is the last day of the month, will be displayed.
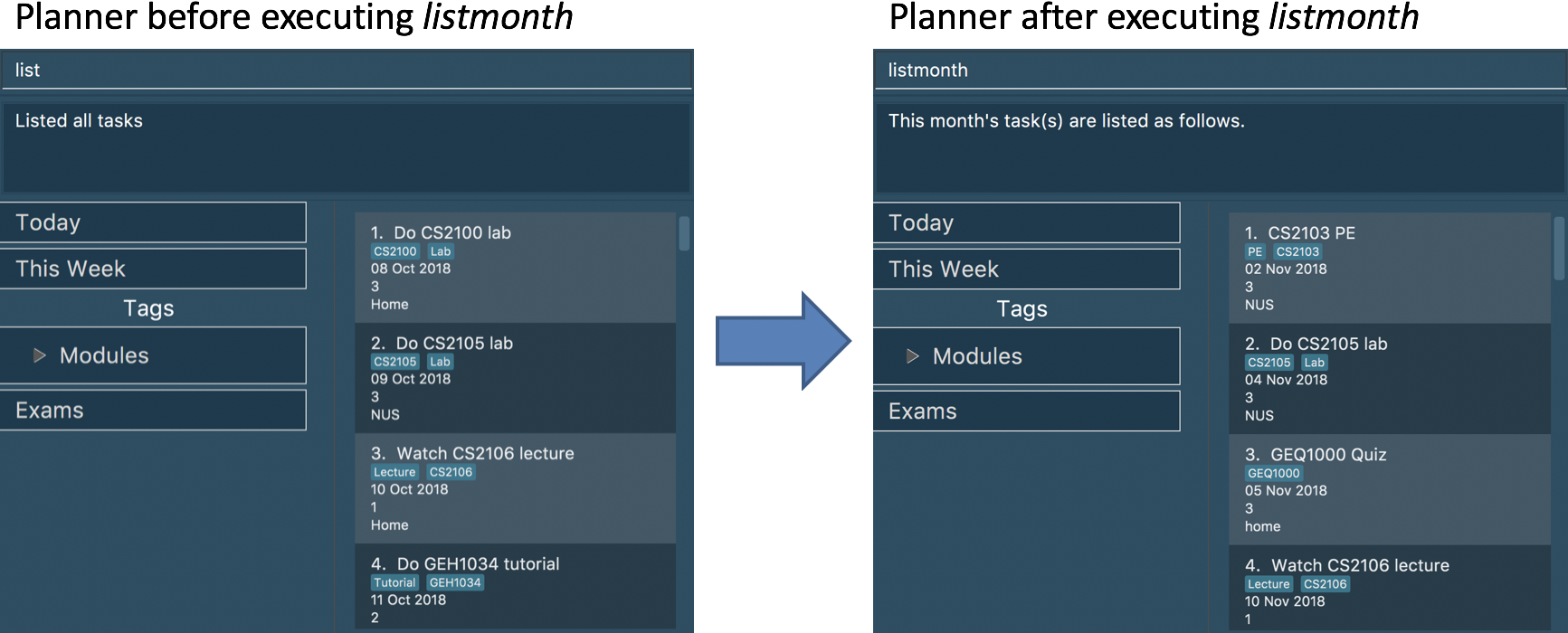
Tasks from 02 Nov '18 to end of the month (30 Nov '18) are listed.
Filtering Tasks by Tags (Inclusive)
Filter tasks according to tags inclusively. Tags matching ANY of those entered by the user will be listed. The filter
is case insensitive. You may include multiple tags when using filter
. For example, filter tutorial quiz project
will return tasks with tags matching at least one of the specified tags.
filter TAG [TAG2] …
Example:
-
filter tutorial
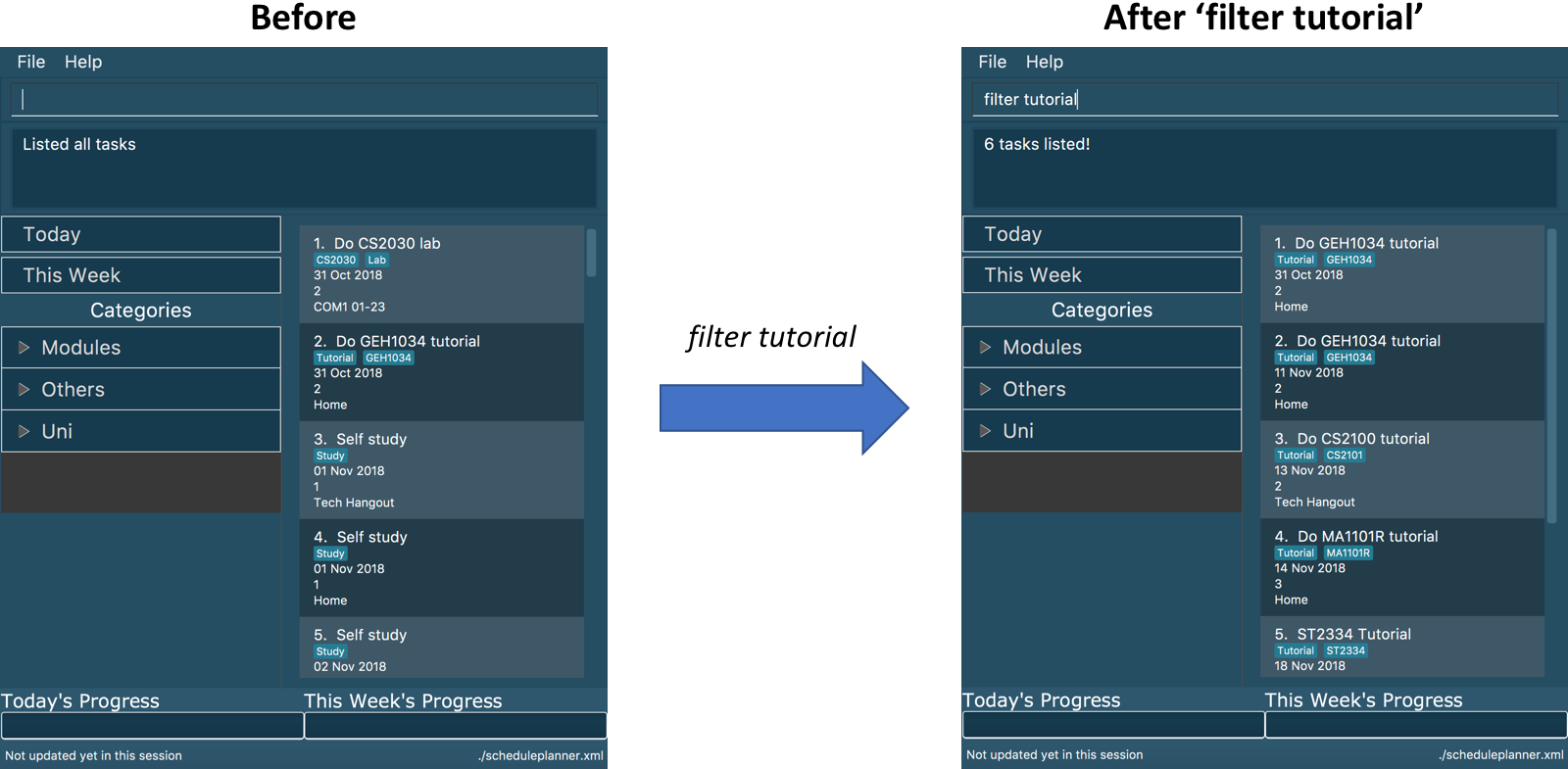
Tasks with the tag tutorial
are listed.
The diagram below illustrates the SSP when filter tutorial
is executed.
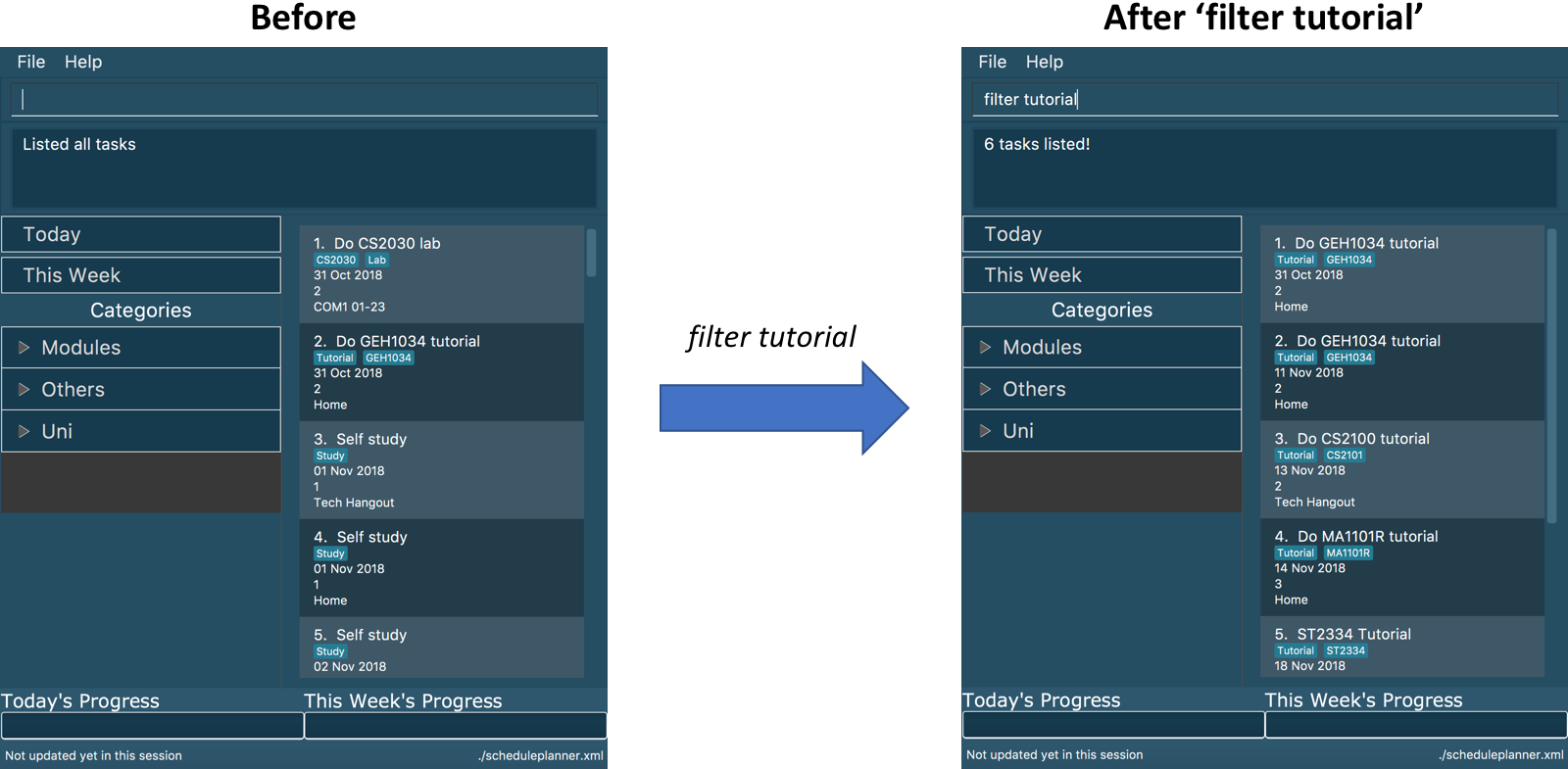
-
filter tutorial cs2100
Tasks with either tutorial
, 2100
tags, or both, are listed.
The diagram below illustrates the SSP when filter tutorial cs2100
is executed.
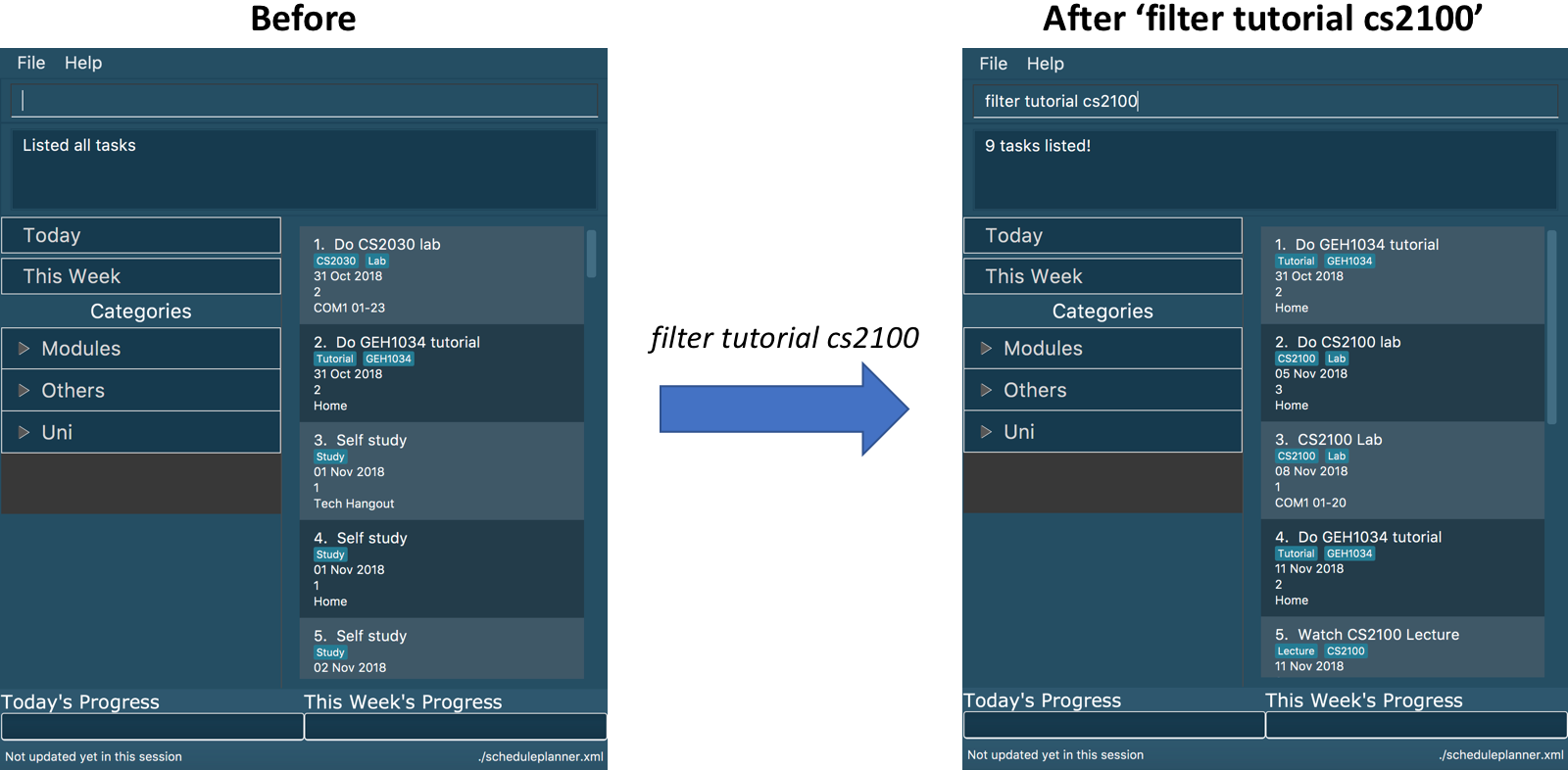
Tasks with either tutorial
, 2100
tags, or both, are listed.
The keyword must be a whole word. E.g for filtering tutorial , tutorial must be used, tut or other variations would not be allowed.
|
The search is case insensitive. e.g apples matches Apples .
The order of the keywords does not matter. For example, filter tutorial cs2103 and filter cs2103
tutorial both return the same tasks. |
Filtering Tasks by Tags (Exclusive)
Filter tasks according to tags exclusively. Tags matching ALL of those entered by the user will be listed. The filter
is case insensitive. You may include multiple tags when using filter
. For example, filter tutorial quiz project
will return tasks with tags matching ALL of the specified tags.
filterstrict TAG [TAG2] …
Example:
-
filterstrict tutorial
Tasks with the tag tutorial
are listed.
The diagram below illustrates the SSP when filterstrict tutorial
is executed.
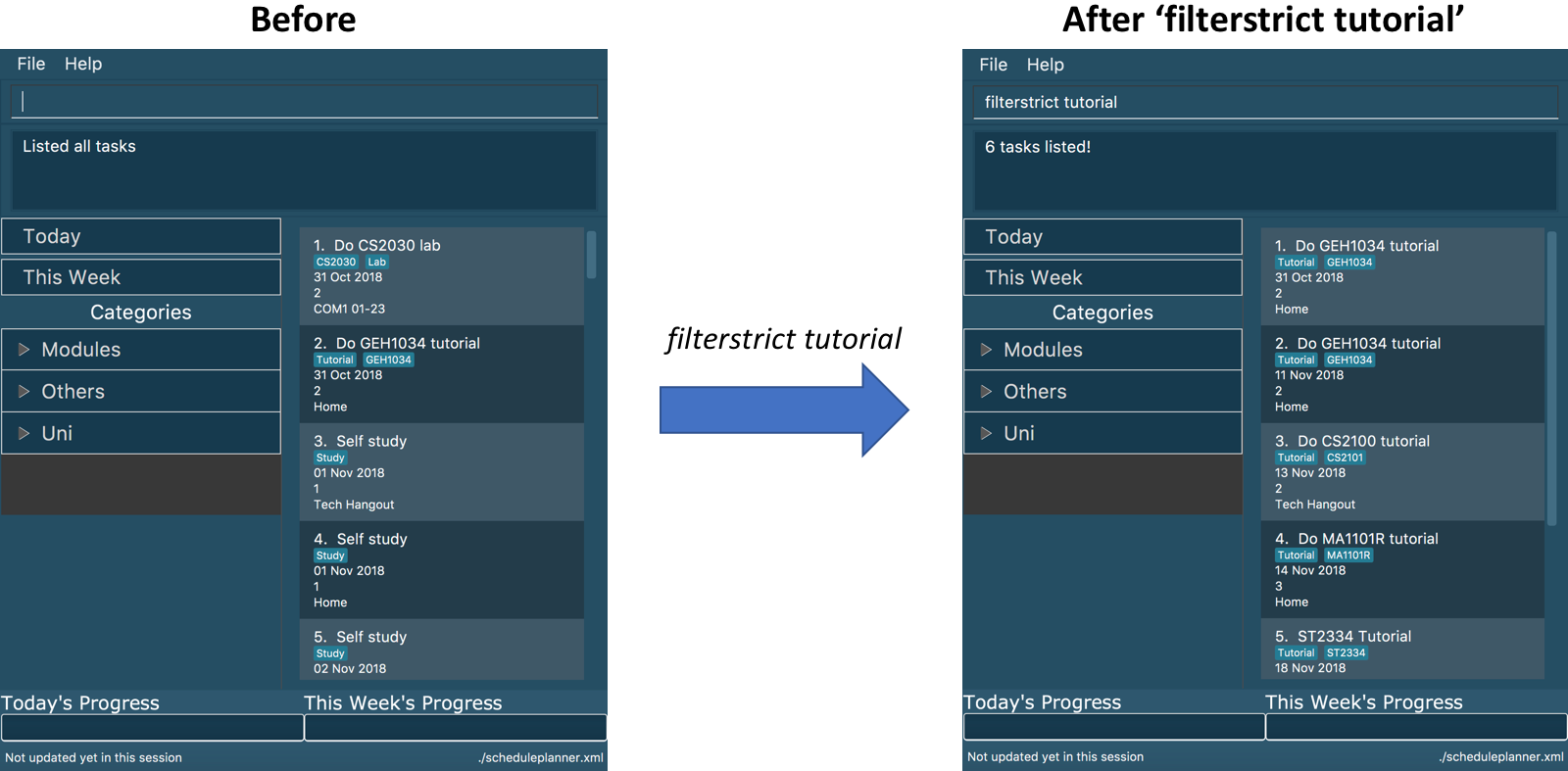
-
filterstrict tutorial geh1034
Tasks with both tutorial
and 2100
tags are listed.
The diagram below illustrates the SSP when filterstrict tutorial geh1034
is executed.
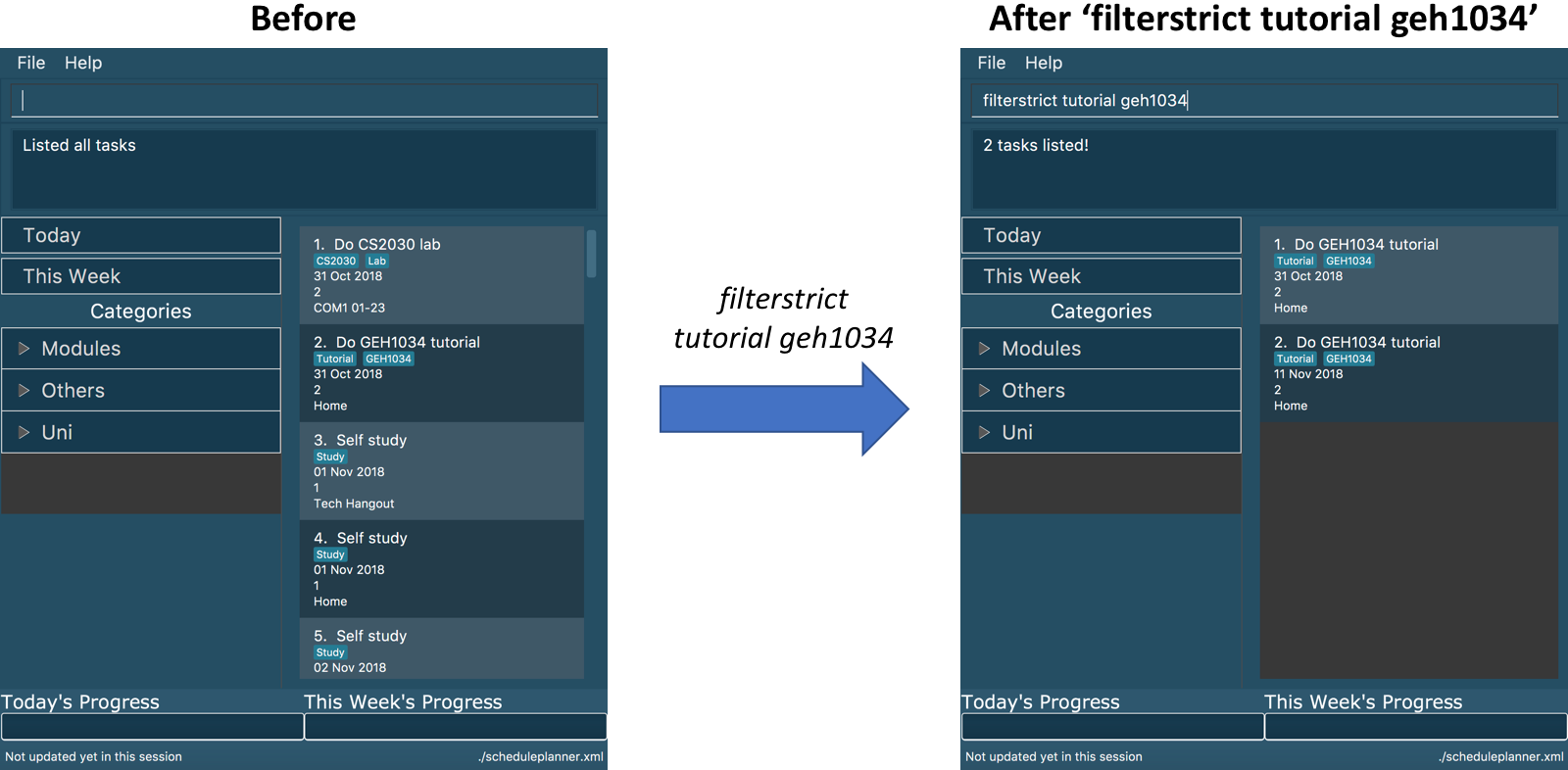
The keyword must be a whole word. E.g for filtering tutorial , tutorial must be used, tut or other variations would not be allowed.
|
The order of the keywords does not matter. For example, filterstrict tutorial cs2100 and [blue]`filterstrict
cs2100 tutorial ` both return the same tasks. |
Contributions to the Developer Guide
Provided below are sections I contributed to the Developer Guide. They showcase my ability to write intelligible technical documentation to aid developers who are looking at the SSP for the first time. It also showcases the technical depth of my contributions to the project, and the value of improvements made to the SSP application. |
List Tasks by Month
The listmonth
command is another variation of the list
command, which supports viewing tasks due from the current
date till the end of the month.
Current Implementation
listmonth
utilises the same implementation used by the list
command:
-
Model#updateFilteredTaskList()
— Takes in a predicate parameter and updates the model according to the predicate.
listmonth
also utilises the same implementation used by the listweek
command:
-
DateWeekSamePredicate
— It takes in adateList
parameter.dateList
is aList<String>
object that contains a list of dates from current date to the end of the month inDDMMYY
format.
listmonth
further implements the following operations:
-
numDaysTillEndOfMonth(currentDay) — It computes the number of days from current date until the end of the month using
currentDay
.currentDay
is the current date inLocalDate
format. -
appendDateList(dateList, numDaysTillEndOfMonth(currentDay)) — It generates sequential
DDMMYY
values based on the result ofnumDaysTillEndOfMonth(currentDay)
and adds them into 'dateList` .dateList
is aList<String>
object.
Given below is an example usage scenario and how listmonth
mechanism behaves at each steps:
Step 1. The user enters the command listmonth
.
Step 2. The command word listmonth
invokes LogicManger
, which invokes SchedulePlannerParser
to return a
ListMonthCommand
object. LogicManager
then invokes ListMonthCommand#execute()
.
Step 3. ListMonthCommand#appendDateList(datelist, numDaysTillEndOfMonth(currentDay))
will be invoked. It
generates sequential dates in DDMMYY
format which are added into dateList
. numDaysTillEndOfMonth(currentDay)
computes the number of days from the current date till the end of the month based on currentDay
. currentDay
is the
current date in LocalDate
format.
Step 4. model.updateFilteredTaskList()
updates the task list with DateWeekSamePredicate
as the predicate.
DateWeekSamePredicate
takes dateList
in Step 3 as its parameter.
Step 5. The updated task list would be reflected on UI
to be displayed to the user.
The following sequence diagram illustrates how the mechanism works:
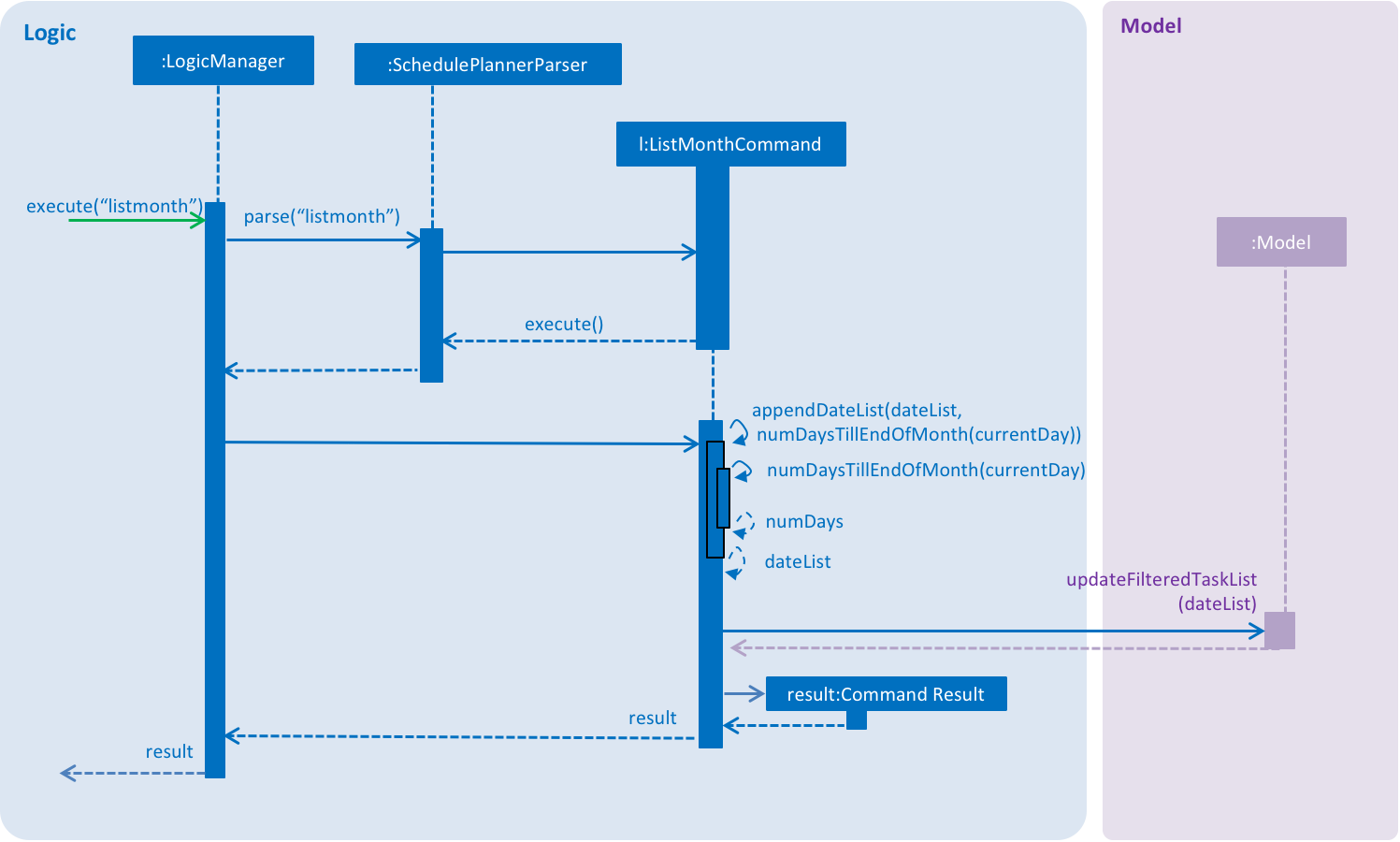
Design Considerations
Aspect: How does listmonth function
-
Alternative 1 (current choice): Fixed date interval based on commands
-
Pros: It is easier to implement, and faster for the user.
-
Cons: It may not be as flexible, as it restricts view to tasks from current date till a fixed date in the future (last day of the month)
-
-
Alternative 2: Allow
list
commands to receive arguments for start/end dates E.g list 130818 200918 to view all tasks between the two dates.-
Pros: It allows flexible viewing for different ranges.
-
Cons: It would be inconvenient to enter two date(s) each time the command is used.
-
Filter by Tags (filter
and filterstrict
)
The filter
& filterstrict
commands allow the user to filter tasks in the schedule planner according to their tags.
The user may search for multiple tags at once, and the schedule planner returns a list of tasks containing the tags
specified by the user.
The filter
command searches for tasks inclusively, which means that when multiple tags are input, SSP filters tasks
containing ANY of the user-input tags (e.g 'A', or 'B', or both 'A' & 'B').
In contrast, filterstrict
,filters tasks containing ALL of the user-input tags. (e.g ONLY 'A' & 'B')
Current Implementation
Since filter
and filterstrict
are implemented in similar fashion, we will simply refer to filter
.
The filter mechanism utilises FilterCommandParser
to parse the user command into separate tags by invoking the method
FilterCommandParser.parse(args)
, where args
are the tags to be filtered. e.g tag1 tag2
will be parsed into
tag1
and tag2
.
Given below is an example usage scenario and how the filter mechanism behaves at each step:
Step 1. The user executes the command filter tutorial CS2100
Step 2. The 'filter' command invokes FilterCommandParser
, which parses the argument tutorial CS2100
into separate
words tutorial
and CS2100
, and are stored in an array in the predicate TagsContainsKeywordsPredicate
.
Step 3. FilterCommandParser
then returns a FilterCommand
, which contains TagsContainsKeywordsPredicate
, a
predicate which tests for the tags 'tutorial' and 'CS2100'. FilterCommand.execute()
calls model
.updateFilteredTaskList(TagsContainsKeywordsPredicate)
, which returns an updated list of tasks containing any of the
tags input by the user.
filter filters tasks inclusively, while filterstrict filters tasks exclusively.
|
The following sequence diagram summarizes what happens when a user executes a new filter command:
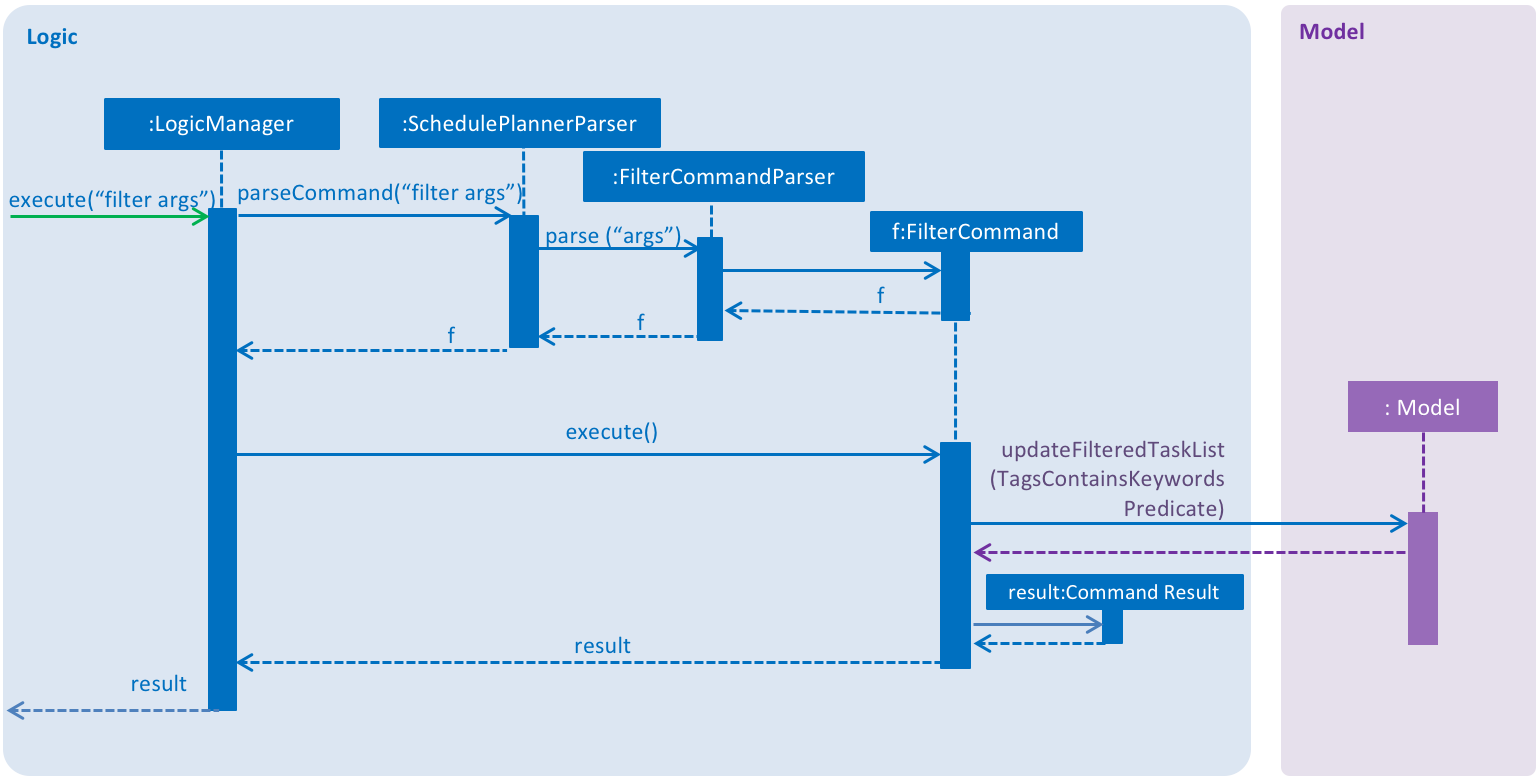
Design Considerations
Aspect: How filter executes
-
Alternative 1 (current choice): The filter command is inclusive i.e filtering for A and B returns tasks with A, B, or both.
-
Pros: It is easy to implement, it is also consistent with how the 'find' command works.
-
Cons: It is not as specific.
-
-
Alternative 2: The filter command is exclusive, i.e filtering for A and B returns tasks with A & B
-
Pros: It is more specific (e.g. filters the tasks more strictly).
-
Cons: It is inconsistent with the original implementation of the 'find' command. It is more difficult to implement.
-